Développer un Service Web REST sous NetBeans
Description
Le service Web de type
REST de cet exercice consiste à fournir le catalogue des formations
de la société FormArmor. Ces données se situent dans la table
Formation de la base de données "FormArmor" sous MySql. Le format
de récupération de la ressource est du XML.
Le service Web sera dans un premier temps testé avec les outils
NetBeans puis "consommer" dans une application cliente
lourde.
Etapes à suivre
Préambule
:
·
Ne
pas oublier d'ajouter le plugin sous Netbeans 12, conformément à la
procédure indiquée dans "Configuration de NetBeans 12" sous le
chapitre Servlet.
Création du
projet Web contenant le Web Service :
·
Démarrer
l'environnement de développement NetBeans.
·
Créer un
nouveau projet File -> New Project... puis Java
Web et choisir Web Application, faire
Next.
·
Dans le nom
du projet choisir le nom WebServiceCatalogue.
·
Comme type
de serveur d'application, choisir Payara Server et laisser
la version Java EE proposée (1.8) puis faire
Next.
·
Ne rien
choisir dans les options de Frameworks, puis faire
Finish. L'initialisation d'un projet Web est en
cours.
Attention
: Si vous
travaillez sur une version >= 12 de Netbeans, ne pas oublier de
modifier les librairies :
Ø
Clic droit
sur Librairies puis propriétés
Ø
Choisir la plateforme JDK 1.8 puis
<OK>
Travail
préparatoire à la création du Web Service :
Ajouter la
librairie (ou le .jar) MySql JDBC Driver.
Dans un
package appelé travail :
·
Création de
la classe Formation (mapping de la table Formation) :
package
travail;
public class
Formation
{
private String libelle;
private String niveau;
private String type;
private String description;
private int diplomante;
private int duree;
private int coutRevient;
public Formation()
{
}
public Formation(String libelle, String niveau, String type, String
description, int diplomante, int duree, int coutRevient)
{
this.libelle = libelle;
this.niveau = niveau;
this.type = type;
this.description = description;
this.diplomante = diplomante;
this.duree = duree;
this.coutRevient = coutRevient;
}
public String getLibelle()
{
return libelle;
}
public void setLibelle(String libelle)
{
this.libelle = libelle;
}
public String getNiveau()
{
return niveau;
}
public void setNiveau(String niveau)
{
this.niveau =
niveau;
}
public String getType()
{
return
type;
}
public void setType(String
type)
{
this.type =
type;
}
public String
getDescription()
{
return
description;
}
public void setDescription(String
description)
{
this.description
= description;
}
public int getDiplomante()
{
return
diplomante;
}
public void setDiplomante(int
diplomante)
{
this.diplomante
= diplomante;
}
public int getDuree()
{
return
duree;
}
public void setDuree(int
duree)
{
this.duree = duree;
}
public int getCoutRevient()
{
return coutRevient;
}
public void setCoutRevient(int coutRevient)
{
this.coutRevient = coutRevient;
}
}
·
Création de
la classe ListeFormations (requêtes SQL vers la table Formation)
:
package
travail;
import
java.sql.*;
import
java.util.ArrayList;
public class
ListeFormations
{
private static ArrayList<Formation> listeFormations = new
ArrayList<Formation>();
private static Connection conn;
private static Statement stmt;
private static ResultSet rs;
private static String pilote =
"com.mysql.cj.jdbc.Driver";
private static String url = new
String("jdbc:mysql://localhost/symfony6_formarmor?useSSL=FALSE&characterEncoding=UTF8");
private static Formation
uneFormation;
public static ArrayList<Formation>
ListeDesFormations()
{
try
{
listeFormations = new ArrayList<Formation>();
Class.forName(pilote);
conn = DriverManager.getConnection(url,"root","");
stmt =
conn.createStatement();
rs = stmt.executeQuery("select * from formation");
while (rs.next())
{
uneFormation = new Formation(rs.getString("libelle"),
rs.getString("niveau"), rs.getString("typeform"),
rs.getString("description"), rs.getInt("diplomante"),
rs.getInt("duree"), rs.getInt("coutrevient"));
listeFormations.add(uneFormation);
}
rs.close();
stmt.close();
conn.close();
return listeFormations;
}
catch
(SQLException e)
{
System.out.println(e.getMessage());
return null;
}
catch
(ClassNotFoundException e)
{
System.out.println(e.getMessage());
return null;
}
}
public static void
creFormation(Formation f)
{
try
{
Class.forName(pilote);
conn =
DriverManager.getConnection(url,"root","");
stmt = conn.createStatement();
String insertion = "('" + f.getLibelle() + "', '" + f.getNiveau() +
"', '" + f.getType() + "', '" + f.getDescription() + "', " +
f.getDiplomante() + ", " + f.getDuree() + ", " + f.getCoutRevient()
+")";
System.out.println(insertion);
int nb = stmt.executeUpdate("insert into formation values" +
insertion);
rs.close();
stmt.close();
conn.close();
}
catch
(SQLException e)
{
System.out.println(e.getMessage());
}
catch
(ClassNotFoundException e)
{
System.out.println(e.getMessage());
}
}
}
Création du
Web Service :
·
Créer un
service Web REST à partir de l'assistant de création Web
Services -> RESTful Web Services from Patterns puis faire
Next.
·
Choisir par
la suite, l'option Simple Root Resource puis faire
Next. Dans le champ Resource Package définir la
valeur jaxws, dans le champ Path saisir la valeur formation,
dans le champ Class Name saisir la valeur Formation, puis
faire Finish.
·
Depuis la
nouvelle classe créée FormationResource, supprimer la méthode
correspondant à la création d'une ressource (méthode
putXml).
·
Dans le
corps de la méthode String getXml() utilisée pour récupérer le
catalogue des formations recopier le contenu suivant
@GET // Méthode HTTP utilisée pour lister le catalogue de
formations
@Produces("application/xml")
public String getXml()
{
ArrayList<Formation> liste =
ListeFormations.ListeDesFormations();
String chaine =
"<listeformation>";
for(int i =0; i < liste.size(); i++)
{
chaine += "<formation>" +
liste.get(i).getLibelle() + "/" + liste.get(i).getNiveau() +
"</formation>";
}
chaine += "</listeformation>";
return chaine;
}
·
Ajouter une
méthode getParamXml() utilisée pour récupérer une formation dont le
libellé est connu :
@GET //
Méthode HTTP utilisée pour afficher une formation dont le libellé
est passé en paramètre
@Path("{lib}")
@Produces("application/xml")
public String getParamXml(@PathParam("lib") String
plibForm)
{
ArrayList<Formation> liste =
ListeFormations.ListeDesFormations();
String chaine = "<listeformation>";
for(int i
= 0; i < liste.size(); i++)
{
if (plibForm.equals(liste.get(i).getLibelle()))
{
chaine += "<formation>" + liste.get(i).getLibelle() + "/" +
liste.get(i).getNiveau() + "/" + liste.get(i).getType() +
"</formation>";
}
}
return (chaine + "</listeformation>");
}
-
Ajouter une
méthode POST creForm() utilisée pour créer une formation dont les
éléments sont passés en paramètres :
@POST //
Méthode HTTP utilisée pour ajouter une formation au
catalogue
//@Produces("application/xml")
public
String creForm(@QueryParam("lib") String plibForm,
@QueryParam("niv") String pnivForm, @QueryParam("typ") String
ptypForm, @QueryParam("des") String pdesForm, @QueryParam("dip")
int pdipForm, @QueryParam("duree") int pdurForm,
@QueryParam("cout") int pcouForm)
{
// A FAIRE : Vérification de la non existence de la
formation
// dont le libellé et le niveau sont passés en
paramètres
Formation maFormation = new Formation(plibForm, pnivForm, ptypForm,
pdesForm, pdipForm, pdurForm, pcouForm);
ListeFormations.creFormation(maFormation);
return plibForm + "/" + pnivForm;
}
- Faire un
Clean and Build à partir du projet WebServiceCatalogue et
s'assurer que le projet se construit correctement.
- Faire un
Deploy à partir du projet. Le serveur Payara doit démarrer.
Sur la console de Payara, un Web Service doit avoir été découvert,
un message similaire doit être affiché :
http://mbaron.developpez.com/tutoriels/soa/developpement-services-web-rest-jaxrs-netbeans/images/glassfishconsole.png
- Ouvrir une
fenêtre d'un navigateur Web et tester la récupération de la
ressource (requête GET via l'URL
http://localhost:8080/WebServiceCatalogue/webresources/formation
Ou
clic droit sur
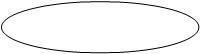
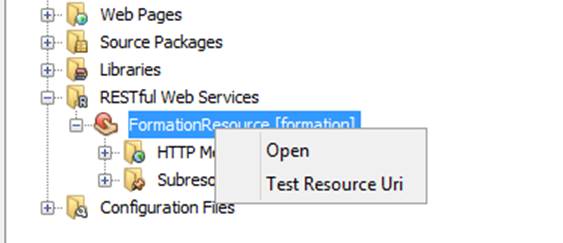
Création de
l’application cliente "consommant" le Web Service :
·
Démarrer
l'environnement de développement NetBeans.
·
Créer un
nouveau projet Java Application et l'appeler
ClientWebServiceCatalogue.
-
Ajouter les
libraires Jax-RS 2.0 et Jersey 2.5.1 et le jar
org.eclipse.persistence.moxy-2.4.2 (fourni).
·
Créer
l'interface graphique voulue
Pour appeler le Web Sercice de type Get et sans paramètre
(affichage de toutes les formations), saisir, à l'endroit voulu, le
code suivant :
Client client =
ClientBuilder.newClient();
String url = "http://localhost:8080/WebServiceCatalogue/webresources/formation";
WebTarget myResource =
client.target(url);
String reponse =
myResource.request(MediaType.APPLICATION_XML).get(String.class);
txtAffichage.setText(reponse);
Ici txtAffichage est une zone de texte de l'interface. "Laisser"
l'IDE importer les packages nécessaires.
Pour appeler le Web Sercice de type Get avec paramètre (affichage
d'une formation choisie), saisir, à l'endroit voulu, le code
suivant :
String url =
"http://localhost:8080/WebServiceCatalogue/webresources/formation/"
+ txtLibForm.getText();
WebTarget myResource =
client.target(url);
String reponse =
myResource.request(MediaType.APPLICATION_XML).get(String.class);
txtAffichage.setText(reponse);
Pour appeler le Web Sercice de type Post avec paramètre (création
d'une formation), saisir, à l'endroit voulu, le code suivant
:
int diplomante;
if (radBtnOui.isSelected())
diplomante = 1;
else
diplomante = 0;
String url =
http://localhost:8080/WebServiceCatalogue/webresources/formation;
WebTarget myResource =
client.target(url)
.queryParam("lib",
txtLib.getText())
.queryParam("niv",
txtNiv.getText())
.queryParam("typ",
txtTyp.getText())
.queryParam("des", txtDes.getText())
.queryParam("dip", diplomante)
.queryParam("duree", txtDur.getText())
.queryParam("cout",
txtCou.getText());
String reponse = myResource.request().post(null,
String.class);
txtAffichage.setText(reponse + " créée");